has been a trusted provider of system software solutions since 1980, specializing in developing powerful, adaptable applications for businesses of all sizes, whether they're starting fresh or evolving existing systems.
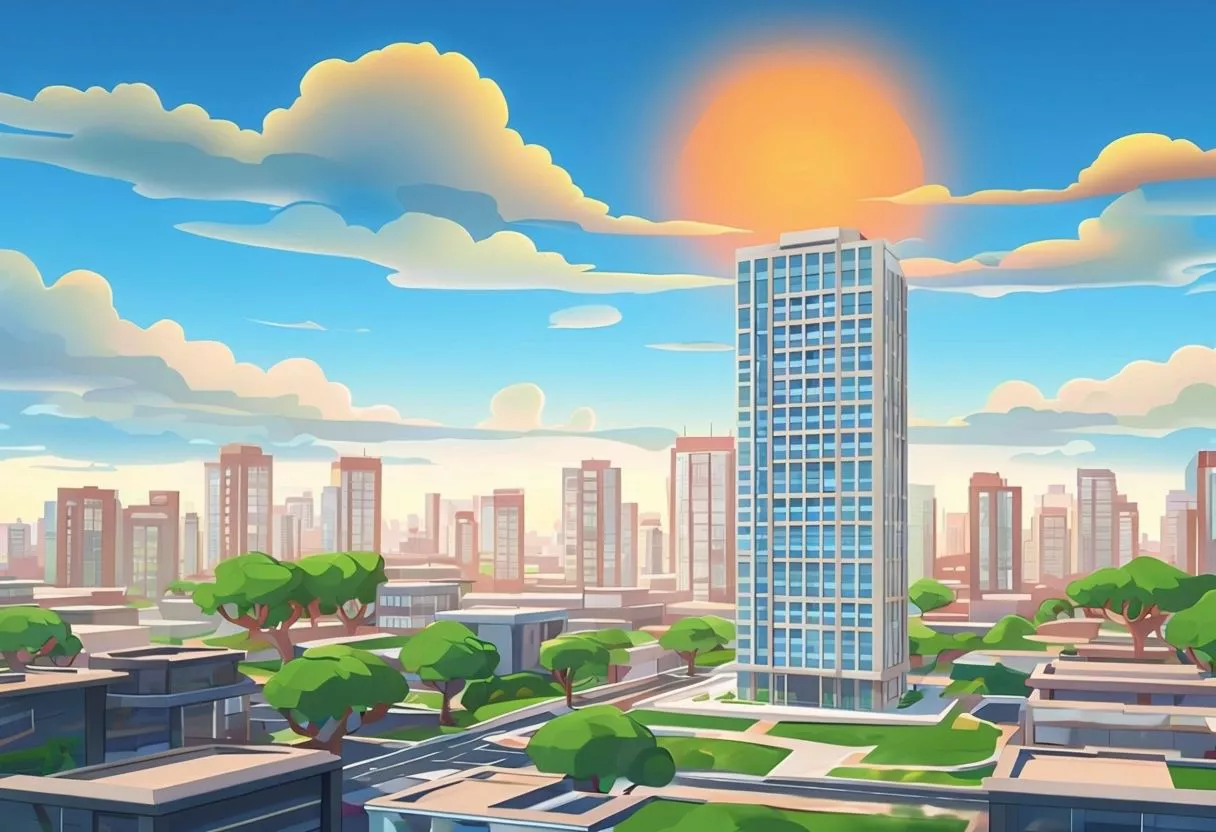
has been a trusted provider of system software solutions since 1980, specializing in developing powerful, adaptable applications for businesses of all sizes, whether they're starting fresh or evolving existing systems.